Best Practices for Nested Data Structures in Syncloop APIs
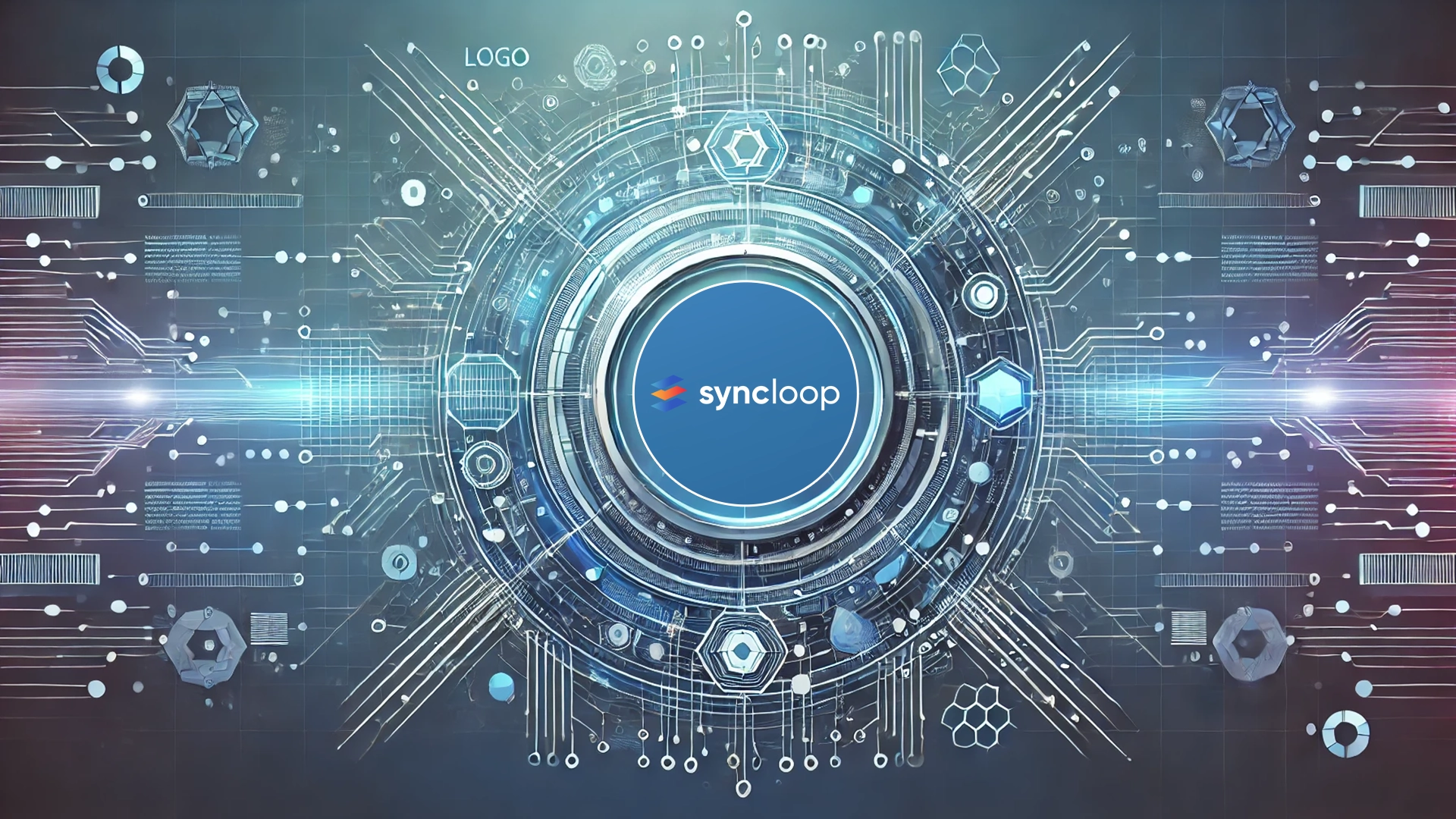
Why Nested Data Structures?
Nested data structures, such as JSON or XML, allow APIs to represent hierarchical relationships, encapsulate complex data, and reduce the number of API calls needed for related information. For example, a nested structure in a product API might include product details, categories, and reviews within a single response.
Best Practices for Nested Data Structures in Syncloop APIs
1. Define Clear Data Schemas
- Use Syncloop's schema tools to define the structure of your nested data.
- Clearly outline parent-child relationships in your data model to avoid confusion or redundancy.
Example: A product schema may define nested objects for details, categories, and reviews.
json
Copy code
{
"product_id": "123",
"details": {
"name": "Laptop",
"price": 1200
},
"categories": ["Electronics", "Computers"],
"reviews": [
{"user": "Alice", "rating": 5, "comment": "Excellent!"},
{"user": "Bob", "rating": 4, "comment": "Great value for money."}
]
}
2. Leverage Transformers for Data Manipulation
Syncloop's transformers can simplify data extraction and manipulation for nested structures. Use transformers to:
- Parse nested objects into flat structures for easier consumption.
- Merge, split, or restructure data to match downstream system requirements.
3. Optimize Query Performance
- Avoid over-nesting, which can lead to performance bottlenecks.
- Use Syncloop’s filtering and pagination features to limit the depth and size of responses, improving API response times.
Tip: Instead of sending deeply nested objects, provide links or references for deeper data retrieval when appropriate.
4. Utilize Error Handling for Nested Structures
- Nested data can introduce complexities in error detection. Use Syncloop's built-in error-handling tools to validate data at each nesting level.
- Define fallback strategies for incomplete or malformed data in nested objects.
5. Document Your API Thoroughly
- Provide comprehensive documentation that describes the nested structure, including all fields, types, and relationships.
- Use Syncloop’s built-in documentation generator to ensure consistency and ease of use for developers.
6. Test and Debug Effectively
- Use Syncloop’s real-time debugging tools to inspect nested data during development.
- Test edge cases, such as empty nested arrays, missing fields, or unexpected data types, to ensure robustness.
7. Design for Scalability
- Ensure your nested structures are future-proof. Anticipate possible changes in data models and use flexible schemas.
- Consider breaking down overly complex structures into modular APIs that interact seamlessly.
Example Use Case: Customer Order API
Scenario: A customer order API requires returning details of an order, including the customer, items, shipping address, and payment details.
Best Practice Implementation:
- Define a nested structure for encapsulating related entities.
- Use Syncloop’s transformers to split or combine data as needed.
- Validate each level of the structure with Syncloop's debugging tools.
json
Copy code
{
"order_id": "456",
"customer": {
"id": "789",
"name": "John Doe",
"email": "john.doe@example.com"
},
"items": [
{"product_id": "123", "name": "Laptop", "quantity": 1, "price": 1200},
{"product_id": "124", "name": "Mouse", "quantity": 2, "price": 50}
],
"shipping_address": {
"street": "123 Main St",
"city": "Metropolis",
"zip": "54321"
},
"payment": {
"method": "Credit Card",
"status": "Paid"
}
}
Conclusion
Nested data structures are powerful tools for API design, but they require careful management to maximize efficiency and usability. By following these best practices, you can leverage Syncloop’s capabilities to handle nested data effectively while ensuring scalability and maintainability.
Generate an image illustrating a hierarchical data structure, with nested objects represented visually, and overlayed tools like transformers and debugging icons to emphasize Syncloop’s capabilities.
Back to Blogs