Designing GraphQL APIs with Syncloop
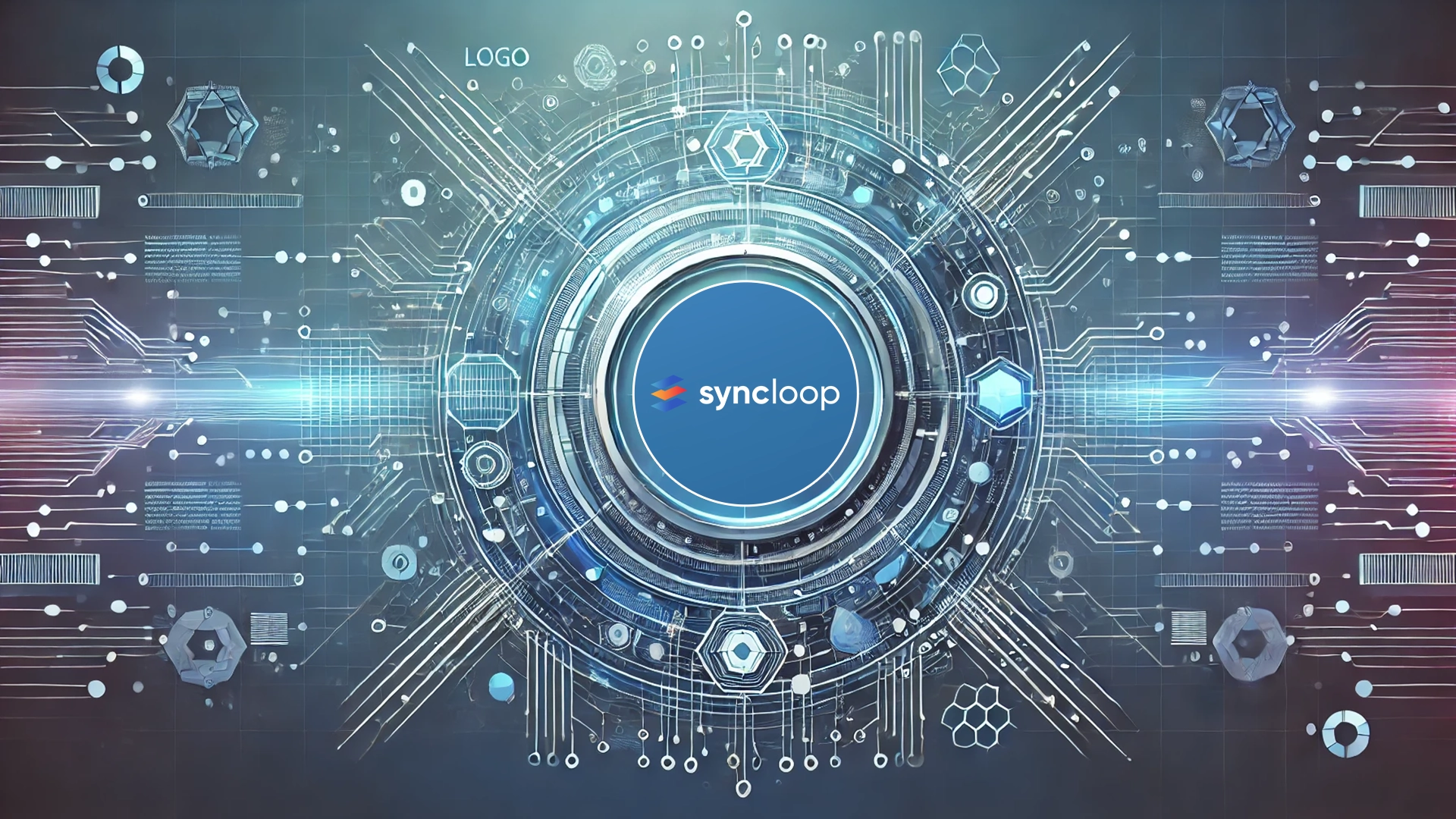
Benefits of GraphQL APIs
- Precise Data Fetching:
- Clients can query only the data they need.
- Reduced Overfetching and Underfetching:
- Streamlines data retrieval and minimizes unnecessary payloads.
- Single Endpoint:
- All requests are handled through a single /graphql endpoint.
- Real-Time Updates:
- Supports subscriptions for real-time data updates.
Syncloop Features for GraphQL API Design
1. GraphQL Schema Builder
- What It Does:
- Provides an intuitive interface to define types, queries, mutations, and subscriptions.
- Benefit:
- Simplifies schema creation and ensures consistency.
2. Real-Time Query Execution
- What It Does:
- Supports live testing of queries and mutations directly within the Syncloop interface.
- Benefit:
- Allows developers to validate functionality during development.
3. Integrated Resolvers
- What It Does:
- Links GraphQL queries and mutations to underlying data sources and logic.
- Benefit:
- Streamlines resolver creation with built-in tools and templates.
4. Subscription Management
- What It Does:
- Enables real-time updates through WebSocket-based subscriptions.
- Benefit:
- Powers features like live feeds, notifications, and real-time collaboration.
5. Analytics and Monitoring
- What It Does:
- Tracks query performance, usage patterns, and error rates.
- Benefit:
- Provides actionable insights to optimize API performance.
Steps to Design GraphQL APIs with Syncloop
Step 1: Define the Schema
- Action:
- Use Syncloop’s schema builder to define types, queries, mutations, and subscriptions.
- Example:
graphql
Copy code
type User {
id: ID!
name: String!
email: String!
}
type Query {
getUser(id: ID!): User
}
type Mutation {
updateUser(id: ID!, name: String, email: String): User
}
Step 2: Configure Resolvers
- Action:
- Map each query, mutation, and subscription to a resolver function.
- Example:
- Resolver for getUser:
javascript
Copy code
const resolvers = {
Query: {
getUser: async (_, { id }) => {
return database.getUserById(id);
},
},
};
Step 3: Test Queries and Mutations
- Action:
- Use the integrated GraphQL playground in Syncloop to test endpoints.
- Example Query:
graphql
Copy code
{
getUser(id: "123") {
name
}
}
Step 4: Implement Subscriptions
- Action:
- Set up WebSocket connections to handle real-time updates.
- Example Subscription:
graphql
Copy code
type Subscription {
userUpdated(id: ID!): User
}
Step 5: Optimize and Monitor
- Action:
- Use Syncloop’s analytics tools to monitor query performance and optimize frequently used queries.
- Example Insights:
- Identify the most queried fields to optimize database indexing.
Best Practices for GraphQL API Design with Syncloop
- Design a Modular Schema:
- Break the schema into smaller, reusable modules for better organization.
- Use Pagination and Filtering:
- Implement these for large data sets to improve performance.
- Rate-Limit Queries:
- Protect APIs from overuse by limiting query complexity and depth.
- Document Your Schema:
- Use auto-generated documentation to guide developers.
- Monitor Performance:
- Regularly analyze query usage to optimize resolvers and underlying databases.
Real-World Applications
- E-Commerce:
- Create APIs for product catalog search, user profiles, and order management.
- Social Media:
- Build APIs for posts, comments, likes, and real-time notifications.
- Healthcare:
- Develop APIs for patient records, appointment scheduling, and live consultations.
- Education:
- Design APIs for course content, assignments, and real-time discussions.
Advantages of Using Syncloop for GraphQL API Design
- Streamlined Development:
- Intuitive tools simplify schema creation and resolver mapping.
- Enhanced Performance:
- Built-in analytics help optimize query execution.
- Real-Time Capabilities:
- Supports subscriptions for interactive features.
- Scalable Solutions:
- Handles large-scale queries and traffic with ease.
- Developer-Friendly:
- Provides a comprehensive playground for testing and debugging.
Conclusion
Designing GraphQL APIs with Syncloop enables developers to build flexible, efficient, and scalable APIs that meet modern application demands. By leveraging Syncloop’s features and following best practices, you can create APIs that deliver exceptional user experiences and empower developers with precise data access.
A conceptual diagram of a GraphQL API architecture built with Syncloop, showcasing schema, resolvers, and real-time subscriptions.
Back to Blogs