Implementing Pagination in APIs with Syncloop
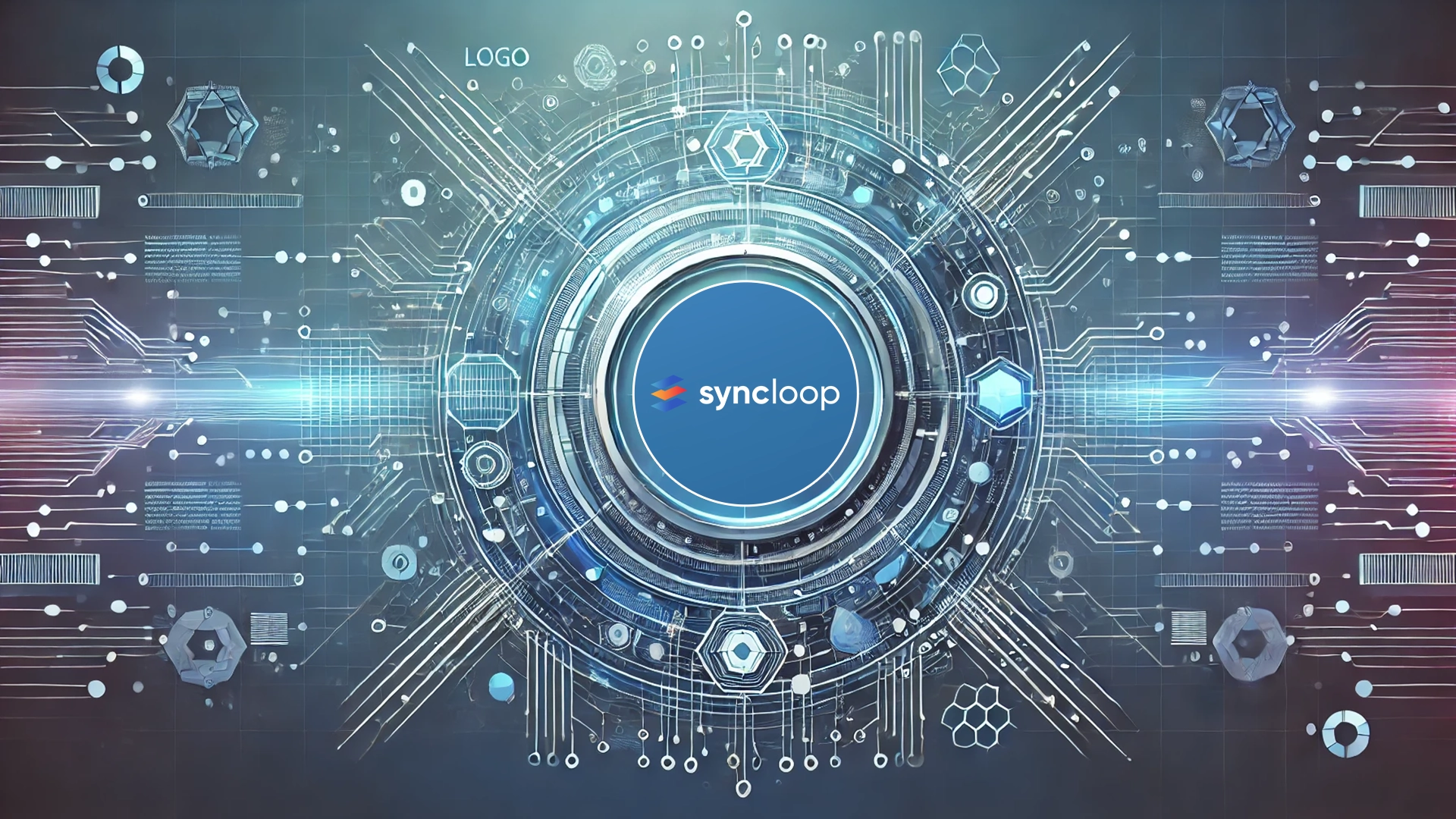
What is Pagination and Why It Matters?
Pagination involves splitting large datasets into smaller pages, which are delivered one at a time through API responses. It is especially useful for:
- Improving Performance: Reduces the load on servers by processing and sending data in smaller parts.
- Enhancing User Experience: Enables faster loading times for applications consuming the API.
- Scalability: Ensures APIs remain responsive even when handling vast amounts of data.
Key Pagination Techniques
- Offset-Based Pagination: Uses an offset and a limit to fetch specific chunks of data.
- Example: GET /items?offset=20&limit=10
- Cursor-Based Pagination: Relies on a unique identifier (cursor) for each record to fetch the next set of results.
- Example: GET /items?cursor=abc123&limit=10
- Page-Based Pagination: Specifies the page number and the number of items per page.
- Example: GET /items?page=3&limit=10
Steps to Implement Pagination in Syncloop
1. Define Pagination Parameters
Start by setting up query parameters like page, limit, or cursor in the API Designer.
- How to Set Up:
- Go to the Syncloop API Designer.
- Add query parameters for offset, limit, or page.
- Specify default values to handle cases where parameters are not provided.
2. Fetch and Process Data
Use Syncloop’s integration tools to fetch data from your database or external service, applying the pagination logic.
- Example:
- For offset-based pagination: SELECT * FROM items LIMIT {limit} OFFSET {offset}.
- For cursor-based pagination: SELECT * FROM items WHERE id > {cursor} LIMIT {limit}.
3. Use Transformers for Data Formatting
Leverage Syncloop Transformers to structure the paginated response, including metadata like total records, current page, and links for navigation.
- Response Format Example:
json
Copy code
{
"data": [...],
"meta": {
"totalRecords": 100,
"page": 1,
"limit": 10,
"nextPage": "/items?page=2&limit=10"
}
}
4. Handle Edge Cases
Account for scenarios where:
- No results are available for the given page.
- The requested limit exceeds the maximum allowed value.
- The cursor or offset is invalid.
- Implementation Tip: Use Syncloop’s Ifelse control to handle such cases and return appropriate error responses.
5. Test the Pagination Workflow
Syncloop provides integrated testing tools to validate the behavior of your paginated API.
- Test Cases:
- Request data with and without pagination parameters.
- Check for proper handling of edge cases like out-of-range pages.
- Verify that pagination metadata is accurate.
6. Optimize for Performance
To ensure efficient pagination, optimize your database queries by:
- Using indexed columns for sorting and filtering.
- Limiting the fields fetched for each record.
- Caching paginated responses when applicable.
Best Practices for Pagination in Syncloop
- Set Default Values: Provide sensible defaults for parameters like limit and page to ensure API usability.
- Include Metadata: Add information like total records, current page, and next/previous links in the response.
- Secure Pagination Parameters: Validate query parameters to prevent SQL injection or excessive data fetching.
- Document Pagination: Clearly specify the pagination format and parameters in your API documentation.
Example: Paginated API Implementation
Request:
http
Copy code
GET /items?limit=10&page=2
Response:
json
Copy code
{
"data": [
{ "id": 11, "name": "Item 11" },
{ "id": 12, "name": "Item 12" },
...
],
"meta": {
"totalRecords": 50,
"page": 2,
"limit": 10,
"nextPage": "/items?page=3&limit=10",
"prevPage": "/items?page=1&limit=10"
}
}
Conclusion
Syncloop makes implementing pagination straightforward, providing tools for data handling, transformation, and response formatting. By leveraging these features, you can enhance your API’s performance and usability, ensuring it handles large datasets efficiently. Start building paginated APIs with Syncloop today to deliver seamless experiences for your users.
An image of a paginated API workflow, showing data retrieval in smaller chunks with page navigation elements, overlaid on a Syncloop interface mockup.
Back to Blogs