Understanding Basic Authentication in Syncloop APIs
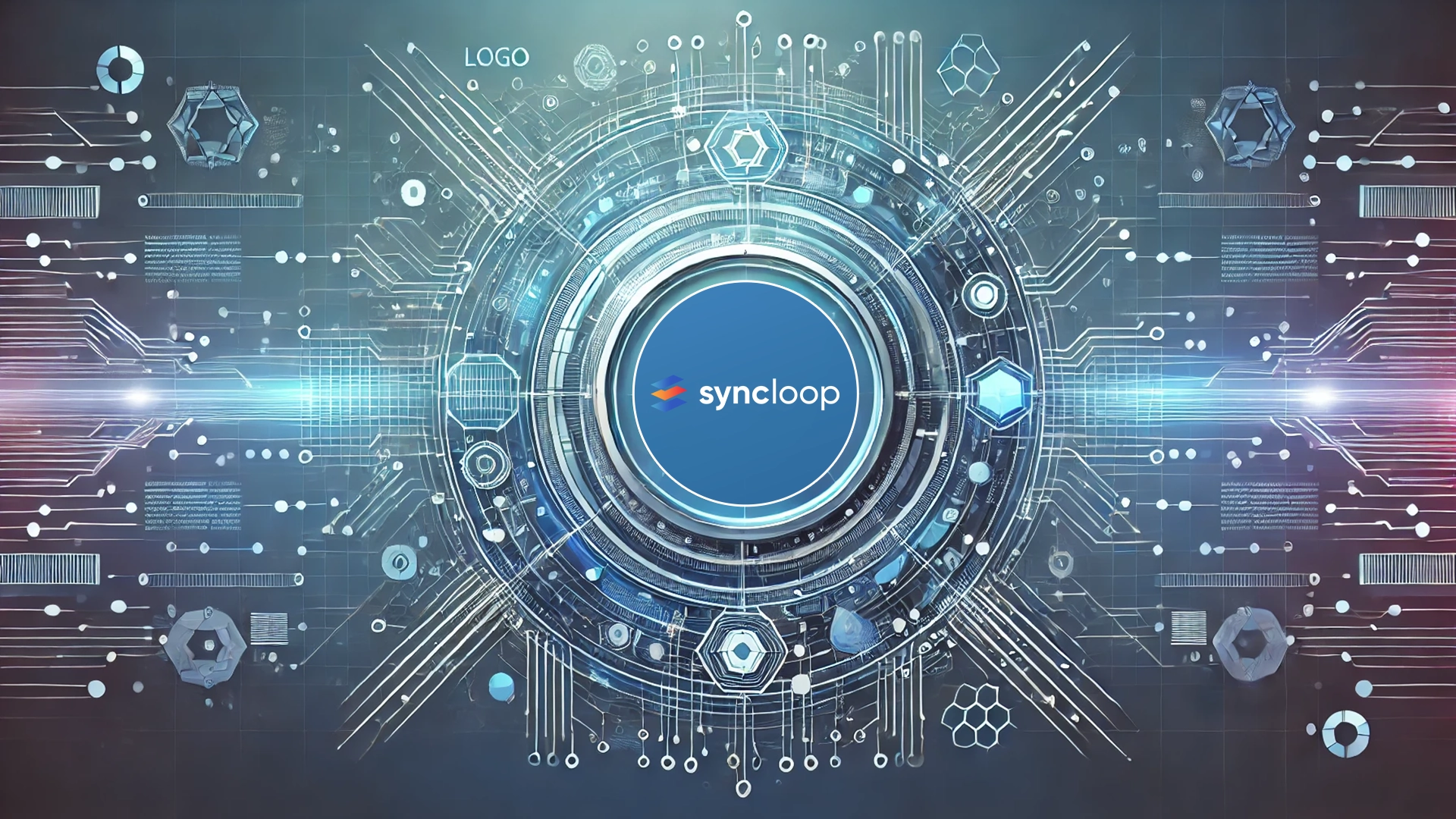
What is Basic Authentication?
Basic Authentication is a method of verifying a user’s identity by encoding credentials (username and password) into an HTTP header. When a client sends a request to an API, the credentials are included in the header for authentication.
How Basic Authentication Works
- Client Request The client sends an HTTP request with a username and password encoded in Base64.
Example Header:
makefile
Copy code
Authorization: Basic dXNlcm5hbWU6cGFzc3dvcmQ=
- Server Validation The API decodes the credentials, validates them against a user database, and grants or denies access.
- Response
- 200 OK: If the credentials are valid, the server processes the request.
- 401 Unauthorized: If invalid, the server rejects the request with an appropriate status code.
Why Use Basic Authentication?
- Ease of Implementation: Simple to set up for small-scale applications.
- Widely Supported: Works seamlessly with most HTTP clients and libraries.
- Secure When Combined with TLS: Encrypting the connection ensures credentials are not exposed in transit.
Implementing Basic Authentication in Syncloop
1. Enable Basic Authentication
- Use Syncloop’s security settings to activate Basic Authentication for specific API endpoints.
2. Define User Credentials
- Store user credentials in Syncloop’s database or integrate with an external authentication service.
- Hash passwords using secure algorithms (e.g., bcrypt) for added protection.
3. Validate Credentials
- Use Syncloop’s transformers to decode the Authorization header and validate the credentials against the database.
Sample Validation Logic:
json
Copy code
{
"decoded_credentials": {
"username": "user1",
"password": "hashed_password"
},
"validation_result": "valid"
}
4. Return Appropriate Responses
- Configure workflows to send 200 OK for valid credentials or 401 Unauthorized for invalid ones.
- Include a WWW-Authenticate header in 401 responses to prompt the client for credentials.
Example Response for Unauthorized Access:
json
Copy code
{
"error": "Unauthorized",
"message": "Invalid credentials"
}
Securing Basic Authentication
- Use HTTPS Always encrypt connections with TLS to protect credentials in transit.
- Hash and Salt Passwords Store only hashed and salted passwords to prevent compromise in case of a database breach.
- Limit Access Restrict API access based on roles or IP addresses where applicable.
- Implement Rate Limiting Prevent brute force attacks by limiting the number of authentication attempts.
- Monitor and Audit Track login attempts and flag suspicious activity using Syncloop’s monitoring tools.
Example Use Case: Securing a Product API
Scenario
A product API requires Basic Authentication to restrict access to authenticated users only.
Implementation Steps
- Define Credentials:
- Store usernames and hashed passwords in Syncloop’s database.
- Activate Authentication:
- Enable Basic Authentication for the /products endpoint.
- Validate Requests:
- Decode the Authorization header and check credentials against the database.
- Handle Responses:
- Return product data for valid requests and a 401 error for unauthorized access.
Sample Request:
http
Copy code
GET /products HTTP/1.1
Authorization: Basic dXNlcm5hbWU6cGFzc3dvcmQ=
Sample Response:
json
Copy code
{
"products": [
{"id": "101", "name": "Laptop", "price": 1200},
{"id": "102", "name": "Smartphone", "price": 800}
]
}
Best Practices for Basic Authentication
- Combine with TLS Ensure all API traffic is encrypted to prevent credential exposure.
- Avoid Storing Plaintext Passwords Use strong hashing algorithms to protect stored passwords.
- Implement Expiry Policies Periodically expire passwords and prompt users to reset them.
- Monitor Failed Login Attempts Track and respond to suspicious login patterns to prevent attacks.
- Educate Users Encourage users to choose strong, unique passwords for added security.
Conclusion
Basic Authentication is a simple yet effective method for securing APIs. Syncloop streamlines its implementation, offering built-in tools and best practices to enhance security and usability. By following these guidelines, you can ensure your APIs are secure, scalable, and easy to manage.
Generate an image showcasing Basic Authentication in Syncloop, with a secure handshake illustration, an HTTP header displaying Base64-encoded credentials, and a server validating access. Use modern and professional design.
Back to Blogs